JavaFXطريقة إظهار Tooltip
المثال التالي يعلمك طريقة إظهار Tooltip لأي شيء تضيفه في النافذة.
مثال
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.PasswordField;
import javafx.scene.control.Tooltip;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage stage) {
// يمثل مربع النص الخاص لإدخال كلمة المرور الذي نريد إضافته في النافذة PasswordField هنا قمنا بإنشاء كائن من الكلاس
PasswordField passwordField = new PasswordField();
// passwordField يمثل الرسالة التي نريدها أن تظهر عند وضع الفأرة فوق الكائن Tooltip هنا قمنا بإنشاء كائن من الكلاس
Tooltip tooltip = new Tooltip("Password should be at least 8 characters");
// passwordField للكائن Tooltip كـ tooltip هنا قمنا بوضع الكائن
passwordField.setTooltip(tooltip);
// في النافذة passwordField هنا قمنا بتحديد مكان ظهور الكائن
passwordField.setTranslateX(100);
passwordField.setTranslateY(110);
// passwordField هنا قمنا بتحديد عرض الـ
passwordField.setPrefWidth(200);
// في النافذة Root Node لأننا ننوي جعله الـ Group هنا قمنا بإنشاء كائن من الكلاس
Group root = new Group();
// root في الكائن passwordField هنا قمنا بإضافة الكائن
root.getChildren().add(passwordField);
// فيها و تحديد حجمها Node كأول root هنا قمنا بإنشاء محتوى النافذة مع تعيين الكائن
Scene scene = new Scene(root, 400, 250);
// هنا وضعنا عنوان للنافذة
stage.setTitle("JavaFX Tooltip");
// أي وضعنا محتوى النافذة الذي قمنا بإنشائه للنافذة .stage في كائن الـ scene هنا وضعنا كائن الـ
stage.setScene(scene);
// هنا قمنا بإظهار النافذة
stage.show();
}
// هنا قمنا بتشغيل التطبيق
public static void main(String[] args) {
launch(args);
}
}
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.PasswordField;
import javafx.scene.control.Tooltip;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage stage) {
// يمثل مربع النص الخاص لإدخال كلمة المرور الذي نريد إضافته في النافذة PasswordField هنا قمنا بإنشاء كائن من الكلاس
PasswordField passwordField = new PasswordField();
// passwordField يمثل الرسالة التي نريدها أن تظهر عند وضع الفأرة فوق الكائن Tooltip هنا قمنا بإنشاء كائن من الكلاس
Tooltip tooltip = new Tooltip("Password should be at least 8 characters");
// passwordField للكائن Tooltip كـ tooltip هنا قمنا بوضع الكائن
passwordField.setTooltip(tooltip);
// في النافذة passwordField هنا قمنا بتحديد مكان ظهور الكائن
passwordField.setTranslateX(100);
passwordField.setTranslateY(110);
// passwordField هنا قمنا بتحديد عرض الـ
passwordField.setPrefWidth(200);
// في النافذة Root Node لأننا ننوي جعله الـ Group هنا قمنا بإنشاء كائن من الكلاس
Group root = new Group();
// root في الكائن passwordField هنا قمنا بإضافة الكائن
root.getChildren().add(passwordField);
// فيها و تحديد حجمها Node كأول root هنا قمنا بإنشاء محتوى النافذة مع تعيين الكائن
Scene scene = new Scene(root, 400, 250);
// هنا وضعنا عنوان للنافذة
stage.setTitle("JavaFX Tooltip");
// أي وضعنا محتوى النافذة الذي قمنا بإنشائه للنافذة .stage في كائن الـ scene هنا وضعنا كائن الـ
stage.setScene(scene);
// هنا قمنا بإظهار النافذة
stage.show();
}
// هنا قمنا بتشغيل التطبيق
public static void main(String[] args) {
launch(args);
}
}
ستظهر لك النافذة التالية عند التشغيل.
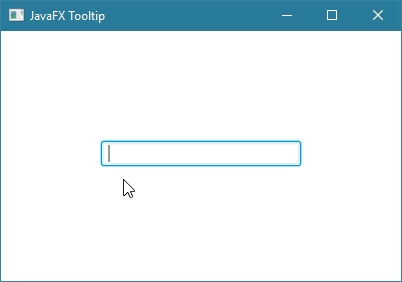