JavaFXطريقة تغيير القيم المعروضة في الـTableView
عند النقر عليها
المثال التالي يعلمك طريقة جعل المستخدم قادر على تغيير القيم الموجودة في الـ TableView
عند النقر عليها.
ملاحظة: هنا كل عامود في الجدول يخزن نوع محدد من البيانات كالتالي:
- عامود الأسماء (Name) يقبل بيانات من النوع
String
. - عامود الأسعار (Price) يقبل بيانات من النوع
Double
. - عامود الكميات (Quantity) يقبل بيانات من النوع
Integer
.
الآن, بما أننا ننوي بناء جدول خاص لعرض المنتجات, سنقوم بتجهيز كلاس إسمه Product
يمثل بيانات أي منتج سيتم عرضه في الجدول.
إذاً الكلاس Product
سيحتوي على ثلاث خصائص و هي الإسم (name
), السعر (price
) و الكمية (quantity
) مع دوال الـ Setters و الـ Getters التي تتيح الوصول لهذه الخصائص.
إنتبه: إن لم تقم بتعريف دوال الـ Setters و الـ Getters في الكلاس Product
تماماً كما فعلنا فإنه لن يظهر لك أي بيانات في الجدول عند تشغيل البرنامج.
تذكر
عند استخدام TableView
لعرض البيانات, فإن JavaFX تتوقع منك أن تقوم بتعريف كلاس خاص تضع فيه عدة خصائص تمثل أسماء و أنواع البيانات التي سيتم تخزينها في كل عامود فيه. كما أنها تجربك على تعريف دوال Setters و Getters مع إختيار التسميات الصحيحة المشتقة من أسماء الخصائص لأنها ستقوم باستدعائهم بشكل تلقائي عند ربط الجدول و أعمدته بهذا الكلاس.
مثال
import javafx.beans.property.SimpleStringProperty; import javafx.beans.property.SimpleDoubleProperty; import javafx.beans.property.SimpleIntegerProperty; public class Product { SimpleStringProperty name; SimpleDoubleProperty price; SimpleIntegerProperty quantity; public Product() { this.name = new SimpleStringProperty(""); this.price = new SimpleDoubleProperty(0.0); this.quantity = new SimpleIntegerProperty(0); } public Product(String name, double price, int quantity) { this.name = new SimpleStringProperty(name); this.price = new SimpleDoubleProperty(price); this.quantity = new SimpleIntegerProperty(quantity); } public String getName() { return name.getValue(); } public void setName(String name) { this.name = new SimpleStringProperty(name); } public double getPrice() { return price.getValue(); } public void setPrice(double price) { this.price = new SimpleDoubleProperty(price); } public int getQuantity() { return quantity.getValue(); } public void setQuantity(int quantity) { this.quantity = new SimpleIntegerProperty(quantity); } }
import javafx.application.Application; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.control.TableColumn; import javafx.scene.control.TableColumn.CellEditEvent; import javafx.scene.control.TableView; import javafx.scene.control.cell.PropertyValueFactory; import javafx.scene.control.cell.TextFieldTableCell; import javafx.stage.Stage; import javafx.util.converter.DoubleStringConverter; import javafx.util.converter.IntegerStringConverter; public class Main extends Application { public void start(Stage stage) { // يمثل الجدول الذي نريد إضافته في النافذة TableView هنا قمنا بإنشاء كائن من الكلاس TableView table = new TableView(); // يمثل مجموعة البيانات التي ستظهر في الجدول Observableهنا قمنا بإنشاء كائن من الكلاس // Product كل سطر في هذا الجدول يمثل كائن من الكلاس ObservableList<Product> data = FXCollections.observableArrayList( new Product("Laptop", 800.00, 3), new Product("Camera", 199.99, 12), new Product("Hard Disk", 79.99, 8), new Product("Projector", 240.55, 7), new Product("PC Screen", 120.00, 6), new Product("Speakers", 33.00, 10), new Product("Headphones", 12.50, 4), new Product("Microphone", 24.75, 5) ); // فقط String هنا قمنا بإنشاء عامود الأسماء و حددنا أنه يقبل قيم من النوع TableColumn<Product, String> columnName = new TableColumn<>("Name"); // تم إضافته في الجدول Product الموجودة في كل كائن name سيُعرض فيه قيم الـ columnName هنا قلنا أن العامود columnName.setCellValueFactory( new PropertyValueFactory<>("name") ); // String يتيح لك إدخال القيمة الجديدة مع ضمان أن لا يتم قيولها إذا لم تكن من النوع TextField سيتم إظهار columnName عند النقر على أي قيمة موجودة في العامود columnName.setCellFactory(TextFieldTableCell.forTableColumn()); // String سيتم حفظ القيمة الجديدة في حال كان نوعها Enter عند النقر على الزر columnName.setOnEditCommit((CellEditEvent<Product, String> t) -> { ((Product) t.getTableView() .getItems() .get(t.getTablePosition().getRow())) .setName(t.getNewValue()); }); // فقط Double هنا قمنا بإنشاء عامود الأسعار و حددنا أنه يقبل قيم من النوع TableColumn<Product, Double> columnPrice = new TableColumn<>("Price ($)"); // تم إضافته في الجدول Product الموجودة في كل كائن price سيُعرض فيه قيم الـ columnPrice هنا قلنا أن العامود columnPrice.setCellValueFactory( new PropertyValueFactory<>("price") ); // Double يتيح لك إدخال القيمة الجديدة مع ضمان أن لا يتم قيولها إذا لم تكن من النوع TextField سيتم إظهار columnPrice عند النقر على أي قيمة موجودة في العامود columnPrice.setCellFactory(TextFieldTableCell.forTableColumn(new DoubleStringConverter())); // Double سيتم حفظ القيمة الجديدة في حال كان نوعها Enter عند النقر على الزر columnPrice.setOnEditCommit((CellEditEvent<Product, Double> t) -> { ((Product) t.getTableView() .getItems() .get(t.getTablePosition().getRow())) .setPrice(t.getNewValue()); }); // فقط Integer هنا قمنا بإنشاء عامود الكميات و حددنا أنه يقبل قيم من النوع TableColumn<Product, Integer> columnQuantity = new TableColumn<>("Quantity"); // تم إضافته في الجدول Product الموجودة في كل كائن quantity سيُعرض فيه قيم الـ columnQuantity هنا قلنا أن العامود columnQuantity.setCellValueFactory( new PropertyValueFactory<>("quantity") ); // Integer يتيح لك إدخال القيمة الجديدة مع ضمان أن لا يتم قيولها إذا لم تكن من النوع TextField سيتم إظهار columnQuantity عند النقر على أي قيمة موجودة في العامود columnQuantity.setCellFactory(TextFieldTableCell.forTableColumn(new IntegerStringConverter())); // Integer سيتم حفظ القيمة الجديدة في حال كان نوعها Enter عند النقر على الزر columnQuantity.setOnEditCommit((CellEditEvent<Product, Integer> t) -> { ((Product) t.getTableView() .getItems() .get(t.getTablePosition().getRow())) .setQuantity(t.getNewValue()); }); // قابلة للتعديل table هنا جعلنا بيانات الجدول table.setEditable(true); // table هنا وضعنا الأعمدة الثلاثة في الكائن table.getColumns().addAll(columnName, columnPrice, columnQuantity); // table في الكائن data هنا قمنا بوضع بيانات الكائن table.setItems(data); // في النافذة table هنا قمنا بتحديد حجم الكائن table.setPrefSize(440, 300); // حتى نستغل كل الحجم الذي حجزناه له table هنا قمنا بتحديد عرض كل عامود بداخل الكائن columnName.setPrefWidth(144); columnPrice.setPrefWidth(144); columnQuantity.setPrefWidth(150); // في النافذة table هنا قمنا بتحديد مكان ظهور الكائن table.setTranslateX(10); table.setTranslateY(10); // في النافذة Root Node لأننا ننوي جعله الـ Group هنا قمنا بإنشاء كائن من الكلاس Group root = new Group(); // root في الكائن table هنا قمنا بإضافة الكائن root.getChildren().add(table); // فيها و تحديد حجمها Node كأول root هنا قمنا بإنشاء محتوى النافذة مع تعيين الكائن Scene scene = new Scene(root, 460, 320); // هنا وضعنا عنوان للنافذة stage.setTitle("JavaFX TableView"); // أي وضعنا محتوى النافذة الذي قمنا بإنشائه للنافذة .stage في كائن الـ scene هنا وضعنا كائن الـ stage.setScene(scene); // هنا قمنا بإظهار النافذة stage.show(); } // هنا قمنا بتشغيل التطبيق public static void main(String[] args) { launch(args); } }
ستظهر لك النافذة التالية عند التشغيل.
لتغيير أي قيمة في الجدول عليك أن تضع الفأرة على الجدول ثم تنقر مرة واحدة على الزر Enter.
بعد أن تدخل القيمة الجديدة و تنقر على الزر Enter, سيتم التشييك عليها لمعرفة ما إذا كان يمكن وضعها في الجدول أو لا لأنه لن يتم قبولها إلا إذا كان نوعها مطابق لنوع العامود.
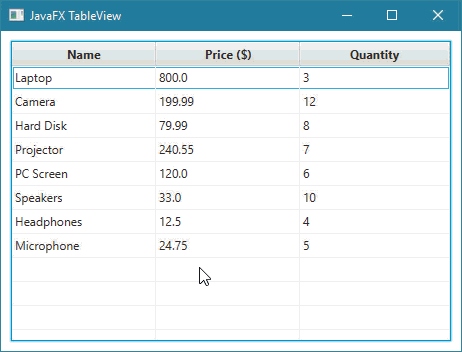